Build Deep Learning model for the Image Classification task (Part1): Pytorch model
The AI, Machine Learning, and Deep Learning definition are becoming viral recently. Let's see how we could apply them to address our problems in the real world, especially in the Computer Vision field for the Image Classification task.
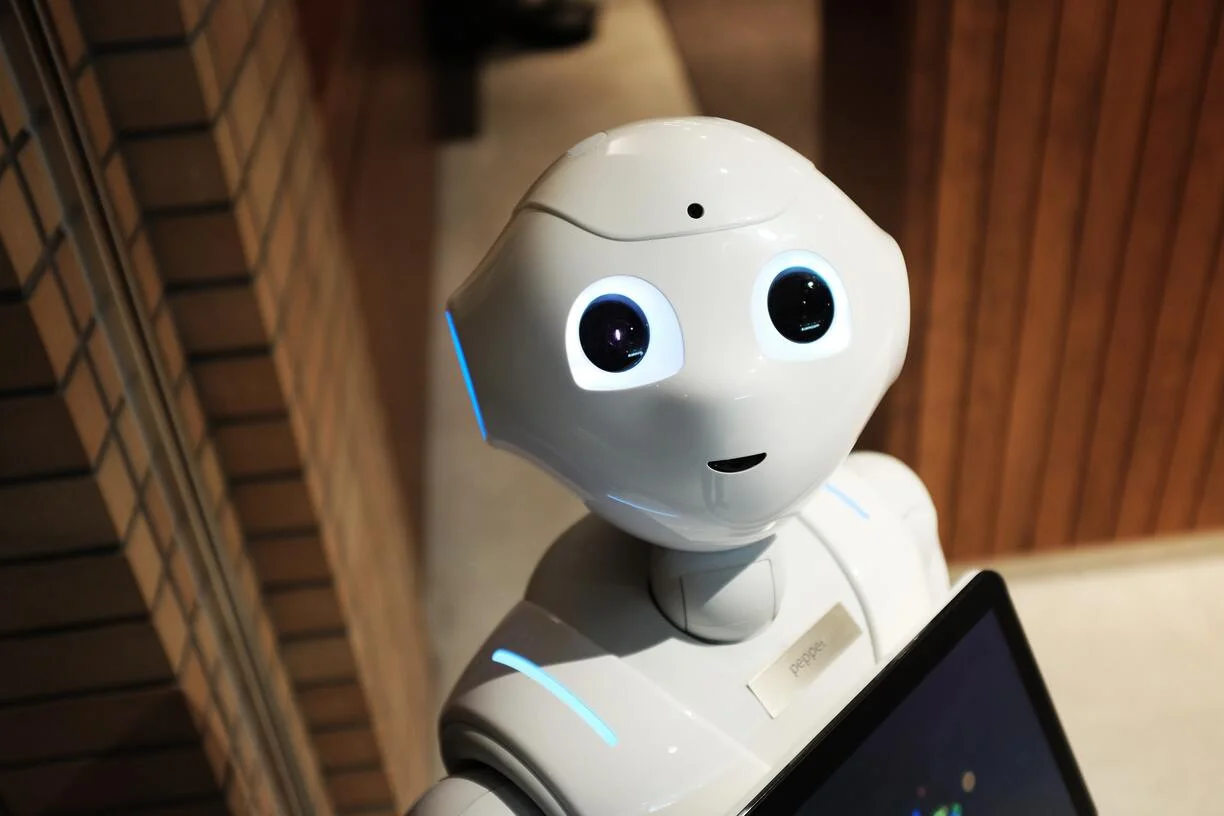
0
Leave a comment
Submit with
Comments (0)