Apply 3d content on a webpage using three.js library
Many individuals wish to build their own 3D website but may feel unsure of where to begin and have the confidence it would run smoothly. If you’re searching for a way to build 3d websites as a developer then this article will 100% help you.
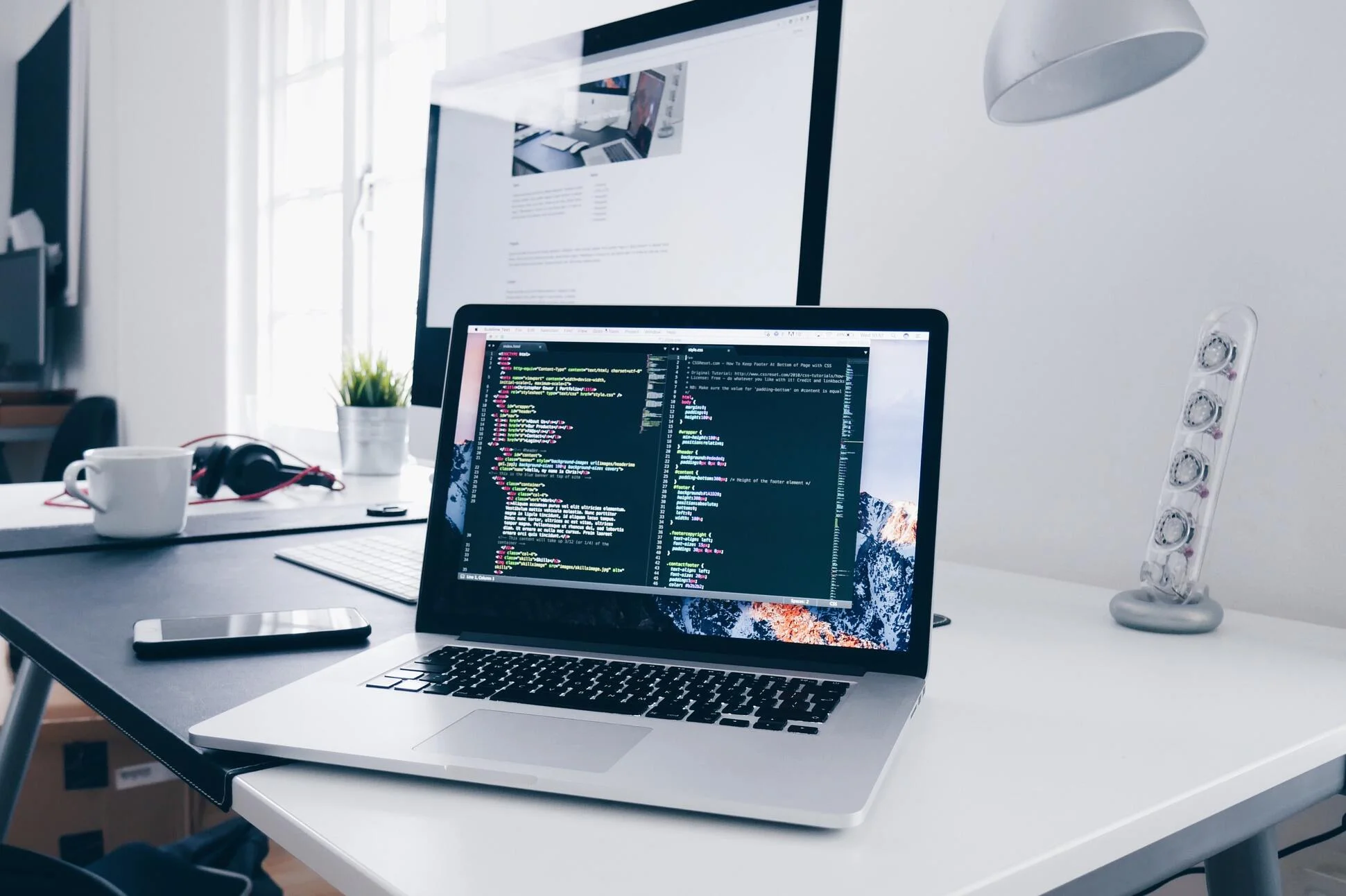
0
Leave a comment
Submit with
Comments (0)